Settings
The model settings are customizable through the user interface. Click the settings button in the toolbar to open the model settings. For clarity, we split the model settings into three separate tabs:
- General
- Graphics
- Physics
These settings are stored on the virtual world and can be controlled via scripting. Please see the API documentation for more details.
General
- Performance Monitor - Toggle to display the performance monitor. The FPS, simulation time, physics time, scripting time, render time (average values and 1% lows), draw calls, triangle count and memory consumption are all recorded.
- View Cube - Toggle to display the view cube.
- Translation Snap - The translation snap factor for the transform gizmo. Hold SHIFT to snap when in translation mode.
- Scale Snap - The scale snap factor for the transform gizmo. Hold SHIFT to snap when in scale mode.
- Rotation Snap - The rotation snap angle for the transform gizmo. Hold SHIFT to snap when in rotation mode.
- Crease Threshold - The crease angle threshold for selecting mesh features. A crease is selectable if the angle between the triangles on both sides of the crease is greater than this threshold.
- Curve Threshold - The curve angle threshold for selecting mesh features. A crease is defined as a set of edges
{e[1], e[2], ..., e[n]}
. An edgee[i]
is in the crease if the angle betweene[i]
ande[i+1]
is less than this threshold.
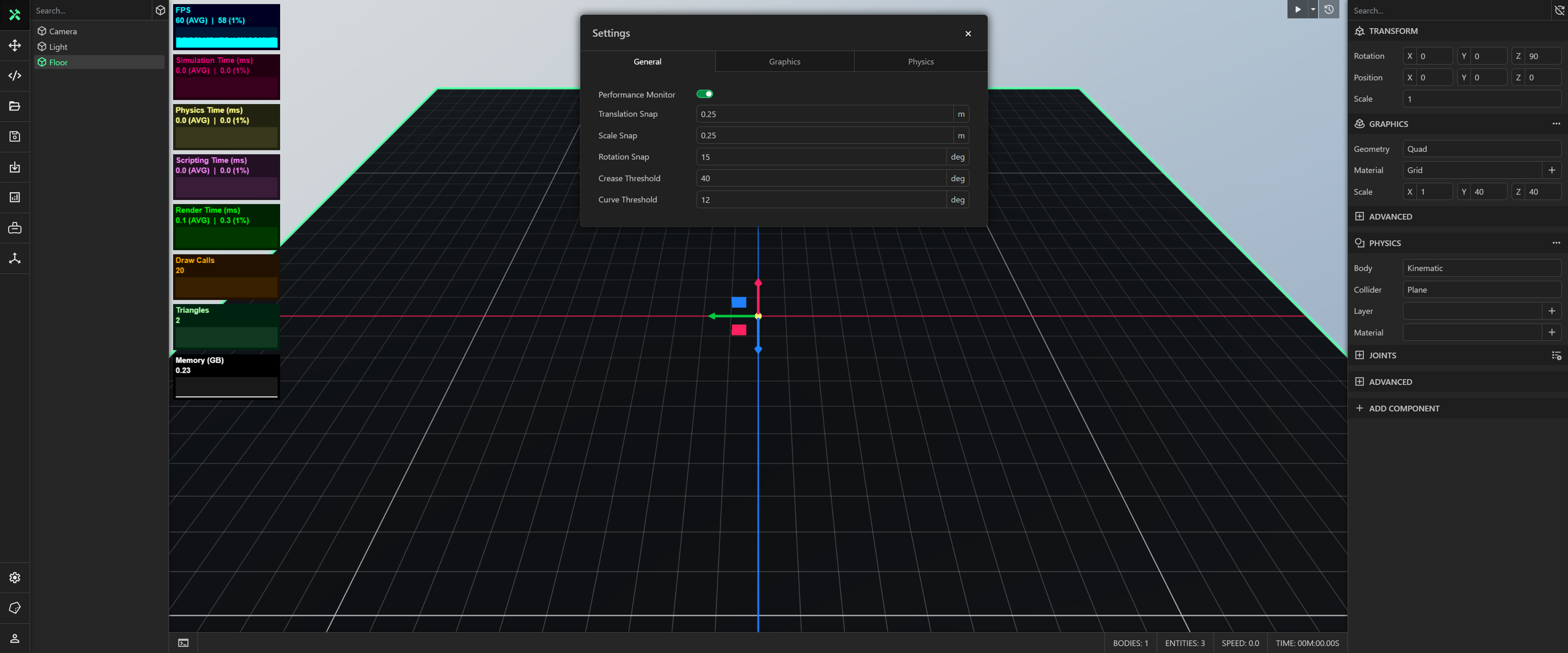
Graphics
- Render Scale - The rendering resolution scale.
- Render Quality - The rendering quality. High quality for PBR and low quality for Blinn–Phong.
- Shadow Quality - The quality of shadows.
- High - PCSS (Percentage Closer Soft Shadows).
- Medium - PCF (Percentage Closer Filtering).
- Low - Hard shadows.
- None - No shadows.
- Ambient Lighting - The lighting constant that is added to the overall lighting of the world to simulate the scattering of light. This ensures that we always give objects in the world some color. Ambient lighting is not necessary if you are using an environment map. It is not used by default.
- Gamma - The gamma parameter controls the gamma correction.
- Brightness - The brightness parameter controls the brightness of the image.
- Environment Map - The environment map are a set of a textures responsible for skyboxes and image-based lighting (IBL). You can use an environment map from our library or import your own HDR environment map by dragging it into the viewport. This will convert your HDR image into three maps:
- Base Map - The cubemap used for the skybox.
- Irradiance Map - The map used for diffuse IBL.
- Radiance Map - The map used for specular IBL.
You may also select custom from the dropdown menu to import your own base map
, irradiance map
and radiance map
textures. You can delete or save these to your desktop.
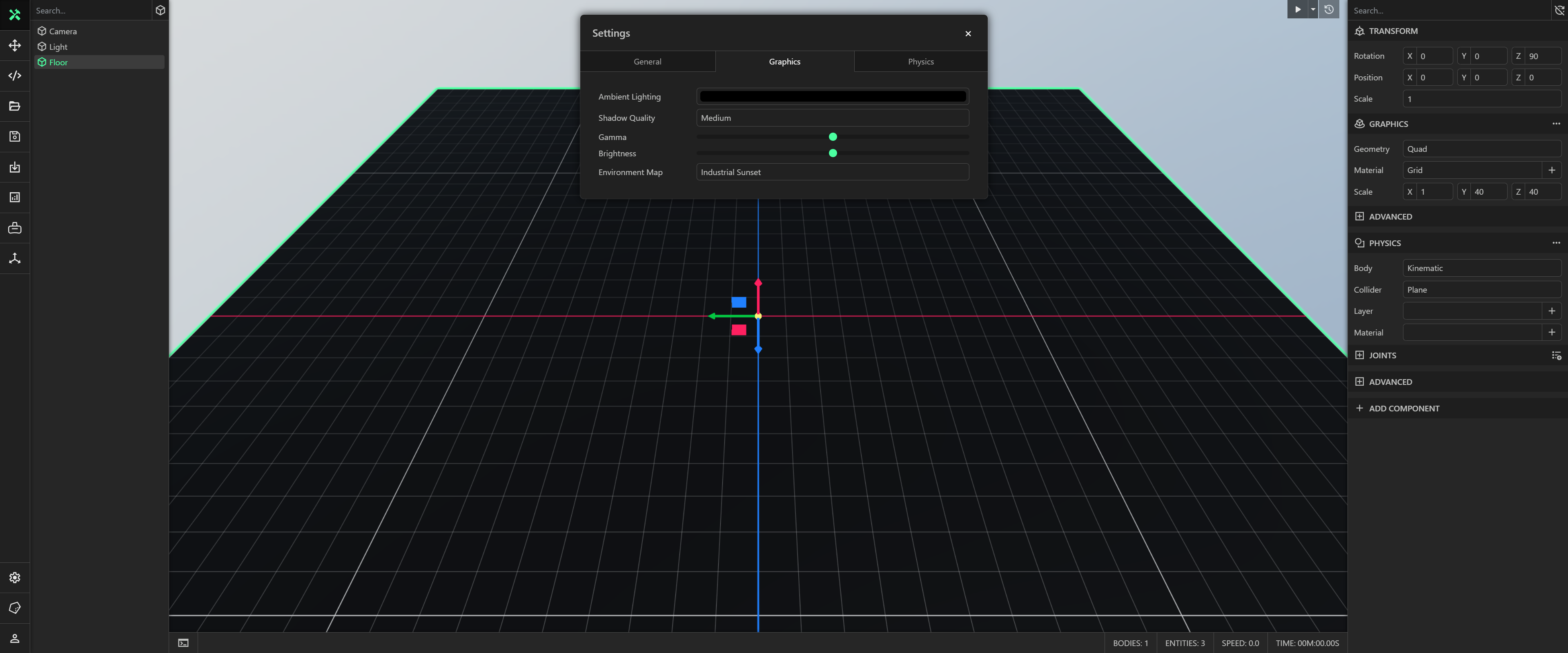
Physics
- Timestep - The unit of time used to advance the state of the physics simulation.
- Solver - The physics constraint solver.
- PGS - Projected Gauss-Seidel.
- TGS - Temporal Gauss-Seidel.
- Default Static Friction - This is the static friction coefficient to use for contacts where one of the colliders does not have a physics material assigned. It is also used for contacts where the static friction coefficient between the pair of physics materials isn’t defined.
- Default Dynamic Friction - This is the dynamic friction coefficient to use for contacts where one of the colliders does not have a physics material assigned. It is also used for contacts where the dynamic friction coefficient between the pair of physics materials isn’t defined.
- Default Restitution - This is the restitution coefficient to use for contacts where one of the colliders does not have a physics material assigned. It is also used for contacts where the restitution coefficient between the pair of physics materials isn’t defined.
- Bounce Threshold - The relative velocity below which restitution is ignored.
- Depenetration Velocity - The maximum velocity that can be used to resolve penetrating physics bodies.
- Position Iterations - The minimum number of position iterations to perform when solving constraints.
- Velocity Iterations - The minimum number of velocity iterations to perform when solving constraints.
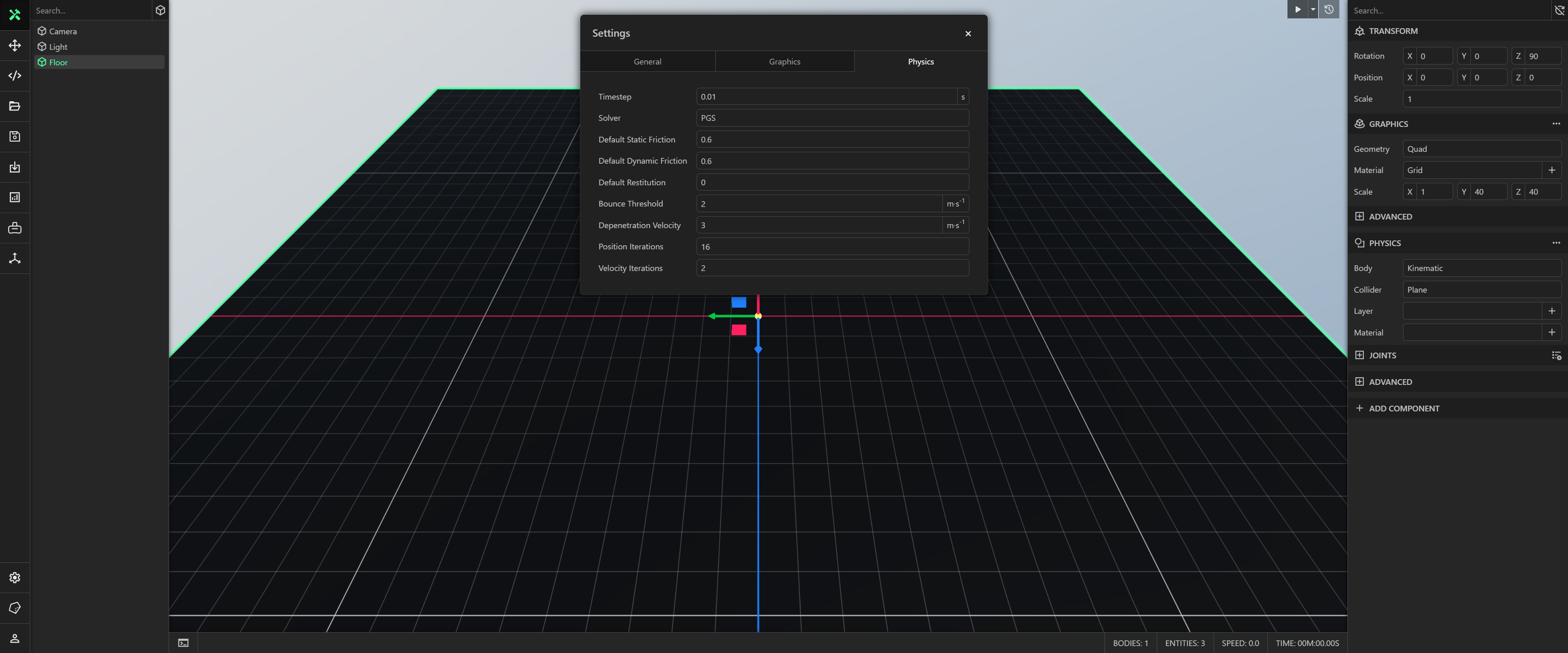
Scripting
The example script below simulates the alternation between day and night by oscillating the brightness
in the settings:
import { Component, type Entity } from "prototwin";
export class Script extends Component {
constructor(entity: Entity) {
super(entity);
}
public override update(dt: number): void {
this.world.settings.brightness = 0.5 * Math.sin(0.25 * this.world.time) + 0.5;
}
}