Bar Series
The Bar Series is a type of plot that represents the relationship between a category and numerical value. A minimal example that records random values between 0 and 100 for each category is provided below:
import { Component, type Entity, Analysis, BarSeries, Random } from "prototwin";
export class Graph extends Component {
#barSeries: BarSeries;
constructor(entity: Entity) {
super(entity);
this.#barSeries = new BarSeries(); // Create the series.
}
public override initialize() {
// Add some categories to the series.
this.#barSeries.add("Palletizer", 0);
this.#barSeries.add("Gantry", 0);
this.#barSeries.add("Robot", 0);
this.#barSeries.add("AGV", 0);
// Create the plot and add the series to it.
const barPlot = Analysis.plots.add("uptime", "Uptime");
barPlot!.add(this.#barSeries);
}
public override update(dt: number) {
const categoryCount = this.#barSeries.x.length;
for (let i = 0; i < categoryCount; ++i) {
this.#barSeries.y[i] = Math.floor(Random.global.nextNumber() * 100); // Assign a random integral value to each category.
}
this.#barSeries.refresh(); // When updating values, we must explicitly refresh for the plot to update.
}
}
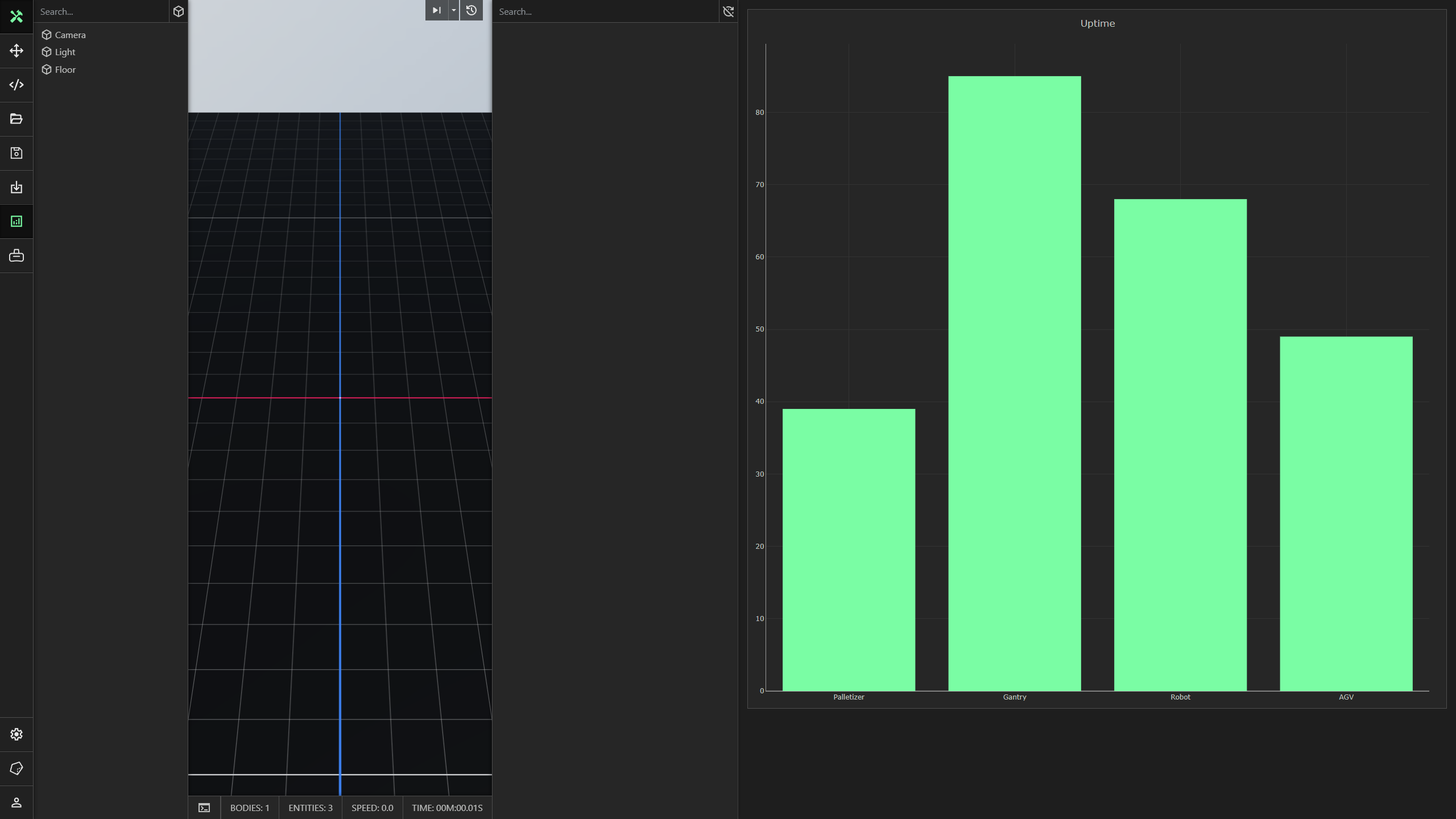