Line Series
The Line Series is a type of plot where sequential data points are connected via straight lines. A minimal example that records the voltage for 3 phases is provided below:
import { Component, type Entity, Analysis, LineSeries } from "prototwin";
export class Graph extends Component {
#phases: LineSeries[];
constructor(entity: Entity) {
super(entity);
this.#phases = [ new LineSeries("Phase 1"), new LineSeries("Phase 2"), new LineSeries("Phase 3") ]; // Create a series for each phase.
}
public override initialize(): void {
// Create the plot and add all the series to it.
const linePlot = Analysis.plots.add("phase", "Three Phase Voltage");
linePlot!.add(...this.#phases);
}
public override update(dt: number): void {
// Add a data point to each phase every timestep.
const t = this.world.time;
this.#phases[0].add(t, Math.sin(t));
this.#phases[1].add(t, Math.sin(t - 2 * Math.PI / 3));
this.#phases[2].add(t, Math.sin(t - 4 * Math.PI / 3));
}
}
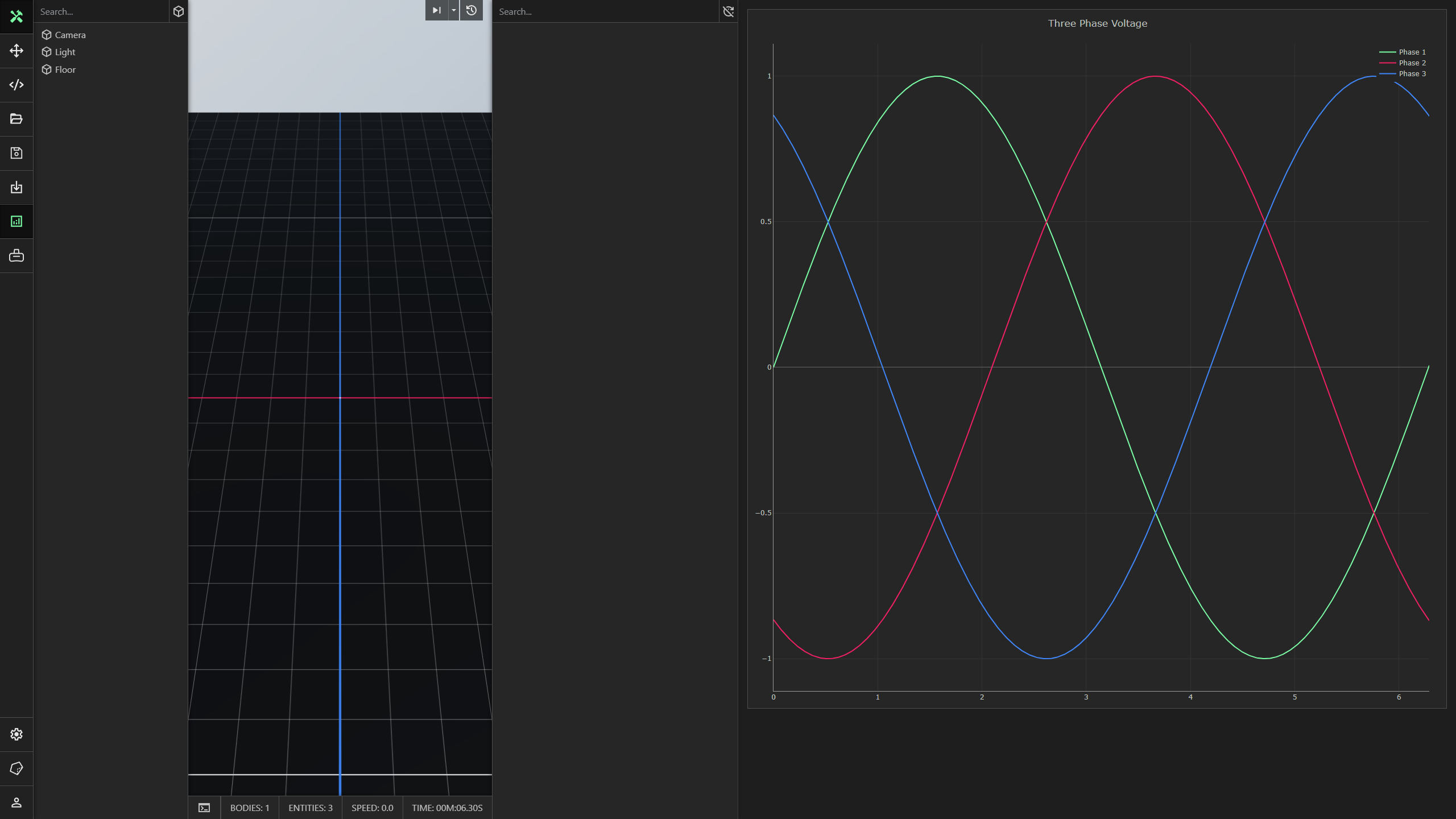