Pie Series
The Pie Series is a circular statistical graphic that is divided into slices to illustrate numerical proportions. A minimal example that records random values between 0 and 1 for each slice is provided below:
import { Component, type Entity, Analysis, PieSeries, Random } from "prototwin";
export class Graph extends Component {
#pieSeries: PieSeries;
constructor(entity: Entity) {
super(entity);
this.#pieSeries = new PieSeries(); // Create the series.
}
public override initialize() {
// Add some slices to the series.
this.#pieSeries.add("Down", 0);
this.#pieSeries.add("Idle", 0);
this.#pieSeries.add("Charging", 0);
this.#pieSeries.add("Active", 0);
// Create the plot and add the series to it.
const pieChart = Analysis.plots.add("state", "AGV State");
pieChart!.add(this.#pieSeries);
}
public override update(dt: number) {
const sliceCount = this.#pieSeries.labels.length;
for (let i = 0; i < sliceCount; ++i) {
this.#pieSeries.values[i] = Random.global.nextNumber(); // Assign a random value to each slice between 0 and 1.
}
this.#pieSeries.refresh(); // When updating values, we must explicitly refresh for the plot to update.
}
}
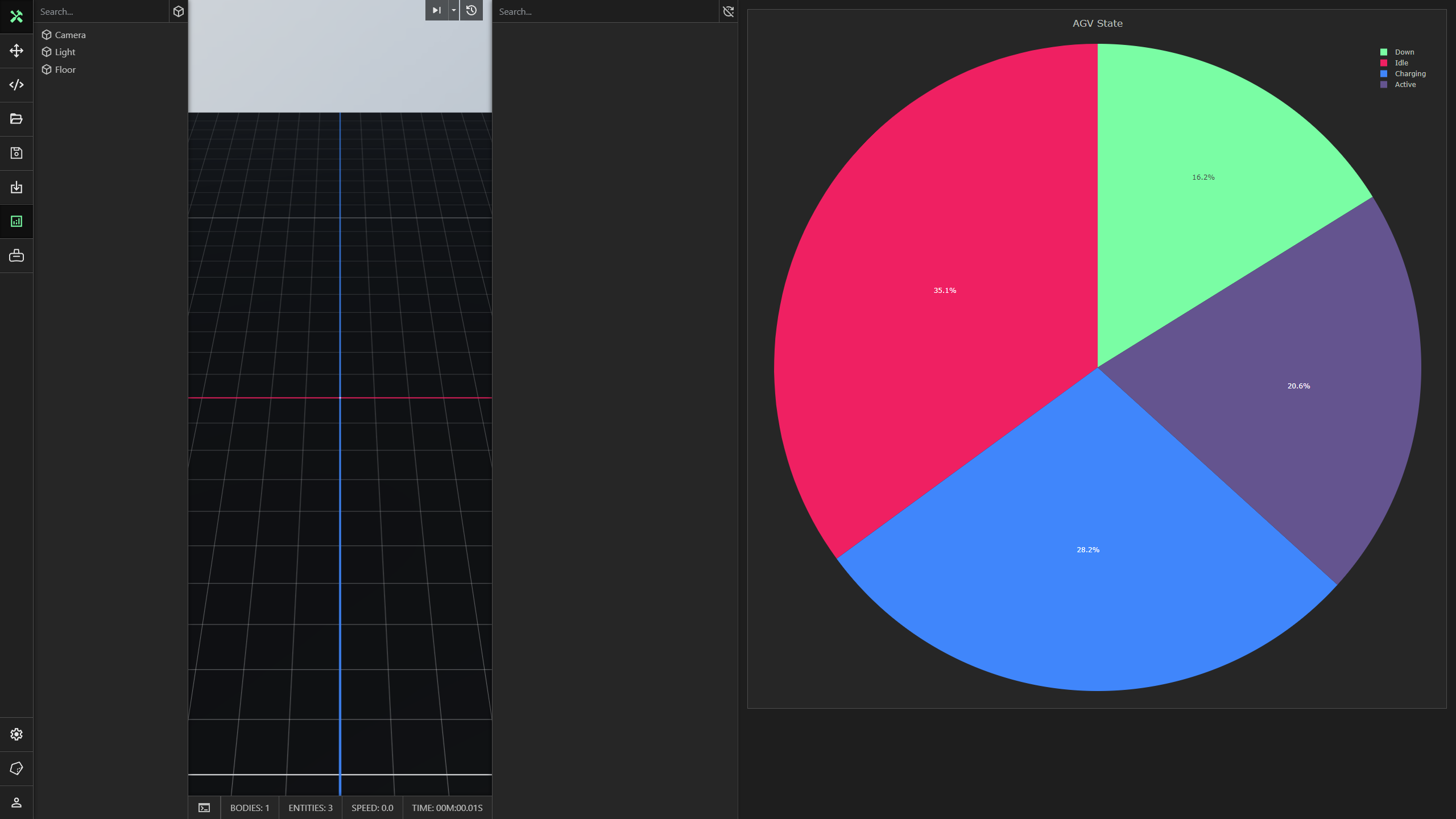